Creating Dynamic Lists and Grids with the React-Virtualized Library
Written on
Chapter 1: Introduction to React-Virtualized
The react-virtualized library allows developers to create virtualized lists that efficiently display large datasets. By integrating it with the AutoSizer component, we can build a list that adjusts its size dynamically based on the viewport. This guide will walk you through the process of setting up auto-resizable lists and grids using this powerful library.
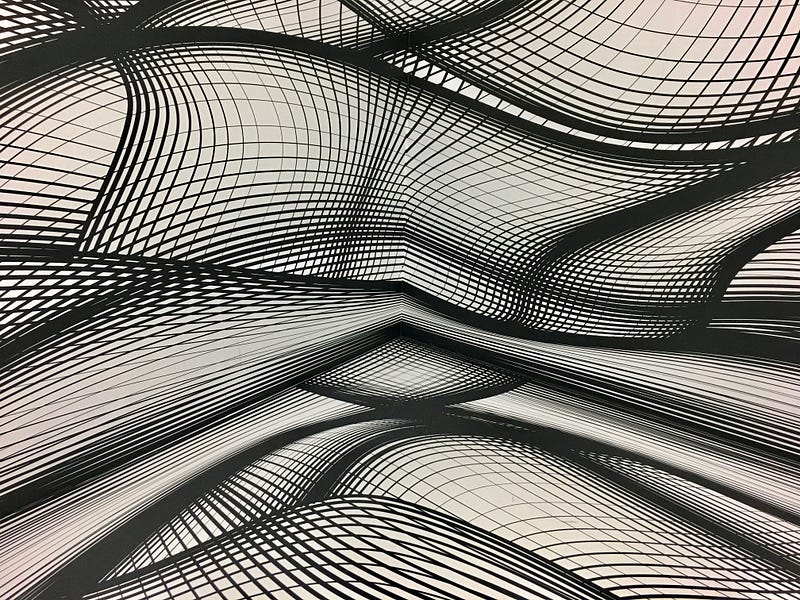
Section 1.1: Installation of React-Virtualized
To get started, you need to install the react-virtualized package. You can do this by executing the following command in your terminal:
npm install react-virtualized
Once installed, you can utilize the AutoSizer component to implement a list that resizes automatically.
Section 1.2: Implementing a Resizable List
Here’s an example of how to create a resizable list:
import React from "react";
import { AutoSizer, List } from "react-virtualized";
import "react-virtualized/styles.css";
const listData = Array(1000).fill(0).map((_, index) => index);
function rowRenderer({ key, index, style }) {
return (
<div key={key} style={style}>
{listData[index]}</div>
);
}
export default function App() {
return (
<div style={{ height: "100vh" }}>
<AutoSizer>
{({ height, width }) => (
<List
height={height}
rowCount={listData.length}
rowHeight={20}
rowRenderer={rowRenderer}
width={width}
/>
)}
</AutoSizer>
</div>
);
}
In this code, we use the AutoSizer to create a list that adjusts its height and width based on the parent container's size. The rowRenderer function is responsible for rendering each item in the list. As you resize your browser window, the list dynamically adjusts its dimensions.
Chapter 2: Creating a Grid with React-Virtualized
The react-virtualized library also allows for the creation of grids. Below is an example of how to implement a grid:
This video demonstrates how to render large lists using react-virtualized or react-window.
Section 2.1: Setting Up the Grid Component
To set up a grid, you can use the following code snippet:
import React from "react";
import "react-virtualized/styles.css";
import { Grid } from "react-virtualized";
let dataList = [];
let nestedArray = [];
let gridWidth = 500;
let gridHeight = 100;
let x;
let y;
for (let i = 0; i < 1000; i++) {
do {
x = Math.floor(Math.random() * gridWidth);
y = Math.floor(Math.random() * gridHeight);
} while (x <= 0 || y <= 0);
if (x > 0 && y > 0) {
nestedArray.push(x);
nestedArray.push(y);
dataList.push(nestedArray);
}
nestedArray = [];
}
function cellRenderer({ columnIndex, key, rowIndex, style }) {
return (
<div key={key} style={style}>
{dataList[rowIndex][columnIndex]}</div>
);
}
export default function App() {
return (
<div>
<Grid
cellRenderer={cellRenderer}
columnCount={dataList[0].length}
columnWidth={100}
height={300}
rowCount={dataList.length}
rowHeight={30}
width={300}
/>
</div>
);
}
In this example, we generate a nested array to hold our grid data. The cellRenderer function is used to render each cell within the grid. By specifying the columnCount, columnWidth, and other properties, we can effectively display our data in a grid format.
This video covers how to render large lists specifically with React Virtualized.
Conclusion: Benefits of Using React-Virtualized
By leveraging the react-virtualized library, developers can create efficient, auto-resizing lists and grids that enhance user experience, particularly when dealing with large datasets. This approach minimizes rendering time and optimizes performance, making it an essential tool for modern web applications.