Creating an Automated Trading Bot with Interactive Brokers
Written on
Understanding Interactive Brokers' TWS
Automated trading has become increasingly popular in the financial markets, allowing traders to execute trades quickly and effectively based on set criteria. This article will guide you through the process of developing a trading bot using the TWS (Trader Workstation) platform from Interactive Brokers, enabling you to automate your trading strategies.
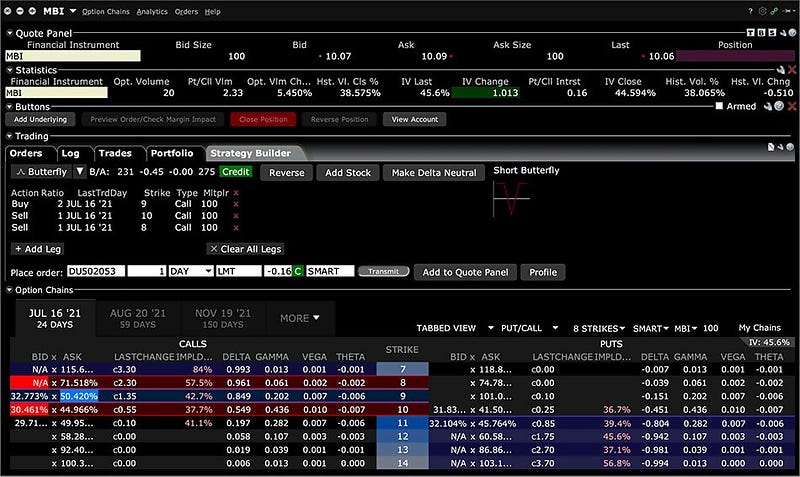
The TWS platform by Interactive Brokers is a robust tool that grants access to a diverse array of financial markets and instruments. It supports extensive algorithmic trading capabilities, such as real-time market analytics, order execution, and account management.
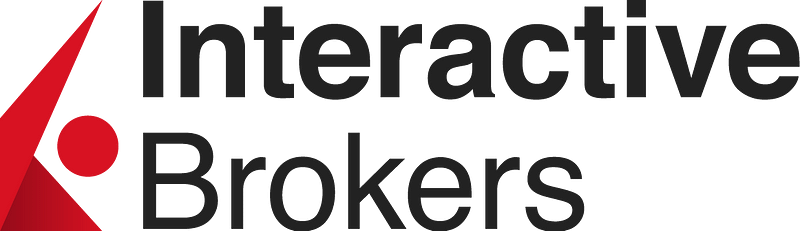
Setting Up Your Development Environment
Before you start coding, ensure that Python is installed on your computer. Additionally, you will need to set up several libraries, including ibapi, ccxt, pandas, and binance. These libraries are essential for communicating with the TWS API, retrieving historical data, and managing account balances.
import ibapi
import queue
from ibapi.client import *
from ibapi.wrapper import *
import threading
from math import *
import pandas as pd
import ccxt
import numpy as np
import time
from binance.client import Client
from ibapi.contract import Contract
from ibapi.order import Order
from ibapi.client import EClient
from ibapi.wrapper import EWrapper
Connecting to the TWS API
The first step in creating our trading bot is to connect to the TWS API. We accomplish this by defining a custom class that inherits from EWrapper and EClient, enabling us to manage server responses and send requests.
class IBapi(EWrapper, EClient):
def __init__(self):
EClient.__init__(self, self)
Creating Order and Contract Objects
Next, we define functions that create order and contract objects. These functions will specify various parameters including the symbol, security type, exchange, currency, quantity, and price.
def make_contract(symbol, sec_type, exch, prim_exch, curr):
contract = Contract()
contract.m_symbol = symbol
contract.m_secType = sec_type
contract.m_exchange = exch
contract.m_primaryExch = prim_exch
contract.m_currency = curr
return contract
def make_order(action, quantity, price=None):
order = Order()
if price is not None:
order.m_orderType = 'LMT'
order.m_totalQuantity = quantity
order.m_action = action
order.m_lmtPrice = price
else:
order.m_orderType = 'MKT'
order.m_totalQuantity = quantity
order.m_action = action
return order
Retrieving Historical Price Data
To make educated trading decisions, it's important to gather historical data. We use the ccxt library to obtain historical price information for a selected financial instrument, such as Bitcoin (BTC) against the US Dollar (USD), from the Binance exchange.
client = Client()
symbol = "BTCUSDT"
klines = client.get_historical_klines(symbol, Client.KLINE_INTERVAL_1DAY, "80 days ago UTC")
df = pd.DataFrame(klines, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume', 'close_time', 'quote_av', 'trades', 'tb_base_av', 'tb_quote_av', 'ignore'])
Implementing Trading Strategies
In this segment, we will implement a straightforward trading strategy based on pivot points. We calculate pivot points and support/resistance levels using historical price data and establish buy and sell conditions based on these levels.
df['close1'] = df['close'].shift(1)
df['open1'] = df['open'].shift(1)
Managing Account Balances
Effective account management is vital in algorithmic trading. We will use the TWS API to retrieve account balances and ensure there are adequate funds (USD) or assets (BTC) available for trading.
ib_api = IBapi()
ib_api.connect("127.0.0.1", 7497, clientId=1)
Executing Buy and Sell Orders
Based on our trading strategy and account balances, we will implement logic to execute buy and sell orders through TWS. This includes specifying order types (market or limit) and dynamically adjusting stop-loss levels to manage risk.
if x == 1:
if Portfolio > 10:
conn = Connection.create(port=7496, clientId=999)
conn.connect()
oid = 1
cont = make_contract('BTC', 'STK', 'SMART', 'SMART', 'USD')
offer = make_order('BUY', 1, 200)
conn.placeOrder(oid, cont, offer)
conn.disconnect()
print("OPEN A LONG", actualPrice)
sl = df.iloc[-2]['close'] * 0.97
time.sleep(5)
else:
pass
Error Handling and Retry Mechanism
To ensure the robustness of our bot, we will implement error handling to catch exceptions and a retry mechanism to attempt failed operations after a brief delay.
try:
# (Your code block here...)
except Exception as e:
print(f"An error occurred: {str(e)}")
time.sleep(300) # Retry after 5 minutes
Conclusion
Developing a trading bot with TWS Interactive Brokers allows traders to automate their strategies and execute trades with accuracy and speed. By following the steps outlined in this article and utilizing Python's capabilities, you can create a powerful trading bot tailored to meet your specific needs and preferences.